ID2116 Week03
OOP is a programming paradigm that models tasks as a collection of cooperating objects and relationships between objects.
Each object containing both data (properties) and functions (methods).
(ref. Smalltalk by Alan Kay)
A kettle, teapot, and cup are all containers that can be filled with liquid and pour it out.
Encapsulation hides internal details - like how a teapot encapsulates both the tea and the ability to pour it.
teapot.pour(cup); // pour tea into a cup
Modularity breaks systems into independent, reusable parts that work together.
let kettle = new Kettle(); let teapot = new Teapot(); let cup = new Cup(); kettle.pour(teapot); // pour water into a teapot teapot.pour(cup); // pour tea into a cup
Hierarchy organizes objects into a hierarchical structure, where each object can inherit properties and methods from its parent.
A class is an abstracted template for creating objects. It defines a set of properties and methods that the objects created from it will have.
How would you organize these fruits in a hierarchy?
What properties and methods do these fruits share?
JavaScripts Objects are defined by key-value pairs. A value can be a text, a number, a function, an object, etc.
let fruit = { //Properties key : value, name: "orange", weight: 100, //Methods weigh() { //function print( this.weight + "g") } }
The dot (.) operator allows you to access an object's properties and methods using the format object.property. For example, if you have an object called fruit, you can access its name property using fruit.name.
fruit.name = "orange"; // set fruit.name to "orange" fruit.weight = 100; // set fruit.weight to 100 fruit.weigh(); // calling fruit's weigh() method // printout "100g"
The this keyword is used to access the object's own properties and methods from within its definition.
let apple = { //key: value color: "red", weight: 100, weigh() { print( this.weight + "g") // this.weight refers to the weight property of the current object } }
A class is a template that defines the properties and methods that all objects created from it will share. Note that no "function" keyword is used for defining methods.
class Fruit { // constructor is a special method that defines the properties of the object constructor(name, weight) { this.name = name; this.weight = weight; this.state = "not peeled" } // method is a function that defines the behavior of the object. weigh() { print( this.name + " weighs " + this.weight + "g"); } }
A class instance is created using the new keyword followed by the class name and any constructor parameters. Here, we create an orange object and an apple object, by passing the name and weight of the fruit to the constructor.
new
// Create an instance of the Fruit class let orange = new Fruit("orange", 100); // Create an orange object let apple = new Fruit("apple", 150); // Create an apple object orange.weigh(); // print out "orange weighs 100g" apple.weigh(); // print out "apple weighs 150g"
let bulb = { //Properties state: "off", //Methods on() { this.state = "on"; }, off() { this.state = "off"; } }
class Bulb { constructor(onImageFile, offImageFile,state) { // properties to store images to show bulb statuses this.bulbOnImg = loadImage(onImageFile); this.bulbOffImg = loadImage(offImageFile); // properties to store state of the bulb this.state = state; } //Switch on the bulb on(){ this.state = "on"; } //Switch off the bulb off(){ this.state = "off"; } }
https://editor.p5js.org/didny/full/ZpW_tMZ0
class Bulb { //Properties constructor(onImage, offImage,state) { // properties to store images to show bulb statuses this.bulbOnImg = loadImage(onImageFile); this.bulbOffImg = loadImage(offImageFile); // properties to store state of the bulb this.state = state; } //Methods on(){ this.state = "on"; } off(){ this.state = "off"; } }
// Setup function runs once when program starts function setup() { createCanvas(400, 400); // Create canvas of size 400x400 pixels bulb = new Bulb("bulb_on.png", "bulb_off.png", "off"); // Create new bulb object with images and initial off state } // Draw function runs continuously in a loop function draw() { background(220); bulb.draw(); // Draw bulb on canvas } // Called when mouse is pressed function mousePressed() { bulb.mousePressed(); // Handle mouse press on bulb }
Look at the state transition diagram of your AA project idea.
List tasks that your agent and user needs to complete as sentences.
For each task, list the actions needed to complete the task as verbs.
For each action, list the objects or things needed to perform that action as nouns.
For each object, list the properties that change as a result of the action as nouns.
Draw a diagram(s) of objects and its properties.
Coding Train: JavaScript Object
Object-Oriented Analysis and Design with Applications
<div style="text-align: center;"> <h1>Variable / Function</h1> </div>
# Light Bulb as an Object 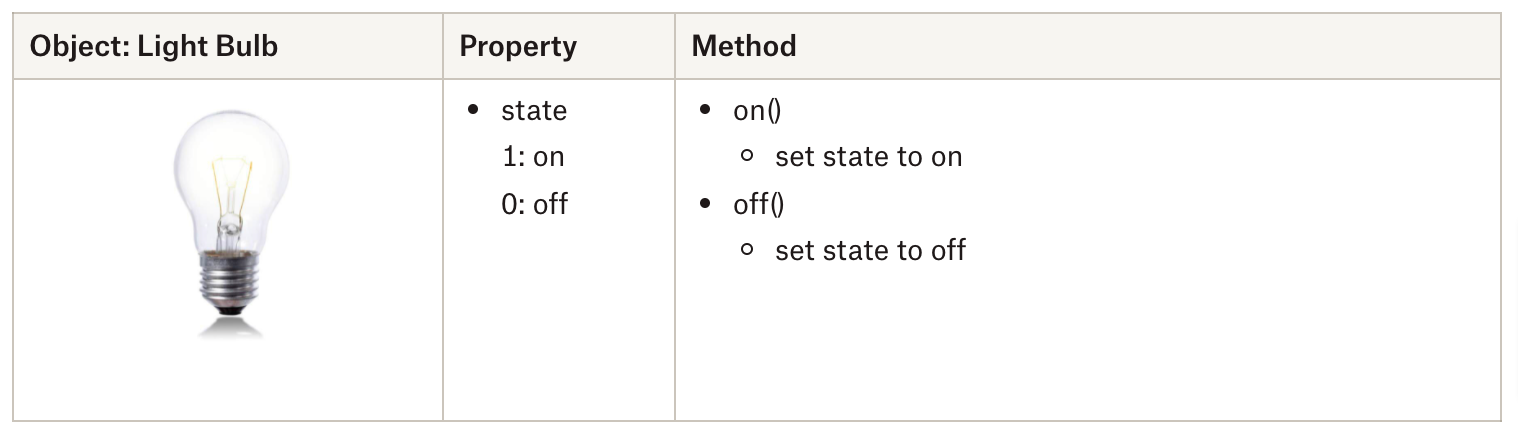 ## Object01: Light Bulb as a JavaScript Object https://editor.p5js.org/didny/sketches/o2xF4PK8f ```js let bulb = { //key: value state: "off", on: function() { this.state = "on"; }, off: function() { this.state = "off"; } } ```